Resource
Resource are full CRUD interfaces for eloquent models and primary feature of Xumina.
Creating Resource
Run following command to create new resource.
php artisan xumina:resource
It will prompt you to select your model.
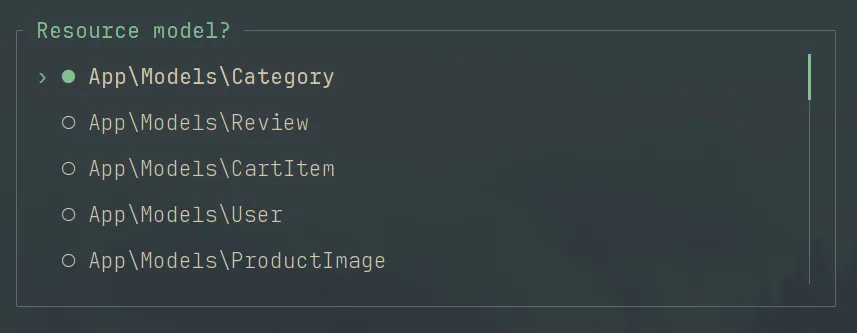
Let’s say you select the Category model, then the command will create following files
Directoryapp
DirectoryXumina
DirectoryAdmin
DirectoryControllers
- CategoryController.php
DirectoryPages
DirectoryCategories
- CreateCategory.php
- EditCategory.php
- ListCategory.php
- ViewCategory.php
DirectoryResources
- Category.php
CategoryController
is exactly like laravel default resource controller with all the same methods (index
, show
, create
, store
, edit
, update
, destroy
). The four resource page that are created are tied to these 7 methods.
- CreateCategory - [
create
,store
] - EditCategory - [
edit
,update
] - ListCategory - [
index
] - ViewCategory - [
show
,destroy
]
Authorization
Resource page use laravel policy class for authorization. Create a policy class for your resource.
Controller methods are mapped to policy class methods in following way
index
:viewAny
show
:view
store
:create
update
:update
destroy
:delete
You can also apply authorization logic inside controller methods.
public function index(Request $request, ListCategory $page): Response { $this->authorize(// your code);
return Inertia::render(Xumina::getInertiaPath($request->route()->getName()), [ 'data' => $page->data(), ]); }